Initial SDK Setup
This guide shows how to add JOIN Stories to your Android application and show your first story in it.
SDK Installation
Our JOIN Stories Android SDK is hosted on Maven Central.
You first need to add it and its dependencies to your app/build.gradle file.
apply plugin: 'com.android.application'
android { ... }
dependencies {
// ...
// JOIN Android SDK
implementation 'com.joinstoriessdk:androidsdk:<latest-version>'
}
plugins {
id("com.android.application")
}
android { ... }
dependencies {
// ...
// JOIN Android SDK
implementation("com.joinstoriessdk:androidsdk:<latest-version>")
}
Tip
Please do not forget to replace latest-version. The latest version is 3.0.0
Warning
JOIN Stories SDK targets Android API level 21 (Android 5.0, Lollipop) or higher.
SDK Initialization
TeamId and widget alias
You will need your team id and the widgets' alias you want to integrate. You can find both of them in the Integration tab of your widget mobile on studio.
To create a widget, check the documentation. Make sure to choose Mobile app environment.
Before using any feature offered by the SDK, you have to initialize it. It is recommanded to do that in Application class.
To do so, you will need a JOIN Stories team ID and then call:
class YourApp : Application() {
override fun onCreate() {
super.onCreate()
JOINStories.init(context: this, joinTeamId: "<your_join_team_id>")
}
}
Init SDK with an api key
You can initialize the SDK with an api key supplied by the JOIN technical team. You can add it to the SDK using the initWithApiKey function :
class YourApp : Application() {
override fun onCreate() {
super.onCreate()
JOINStories.initWithApiKey(context: this, joinTeamId: "<your_join_team_id>", apiKey:"<api_key>")
}
}
Proguard rules
if you're minifying your application, you need to add this rule to accept objects from the JOIN Stories SDK and avoid certain crashes.
-keep class com.joinstoriessdk.androidsdk.internal.data.model.** { *; }
Add Bubble Trigger View
You can add BubbleTriggerView to your app either from XML layout, using the programmatic approach or from Jetpack Compose layout.
<com.joinstoriessdk.androidsdk.ui.BubbleTriggerView
android:id="@+id/join_trigger"
android:layout_width="match_parent"
android:layout_height="wrap_content"
...
/>
val bubbleTriggerView = BubbleTriggerView(context)
view.addView(bubbleTriggerView)
AndroidView(
factory = { context ->
BubbleTriggerView(context)
},
modifier = Modifier
.fillMaxWidth()
)
Warning
To use the widget and the player, the context used must be an activity when the view is initialized.
Integrate in Compose
If you want to integrate a trigger into a Compose view, you need to add this view to the remember function to preserve the state of the widget and avoid destroying and recreating the trigger during Composition.
Destroying and recreating the view will generate a loaded widget, which will be counted towards your pricing.val bubbleTriggerView = remember { BubbleTriggerView(context) } AndroidView( factory = { bubbleTriggerView } )
Initialize Bubble Trigger View
You need to initialise BubbleTriggerView
to take full advantage of your trigger.
bubbleTriggerView.init = JOINStoriesInit(alias: "<your_story_alias>")
Good job !
You have integrated your first trigger and you are ready to see stories in your application.
For a complete integration, see this project : https://github.com/teamjoin/join-stories-sdk-android-demo
Info
You can use others trigger format. For more info : UI Customizations
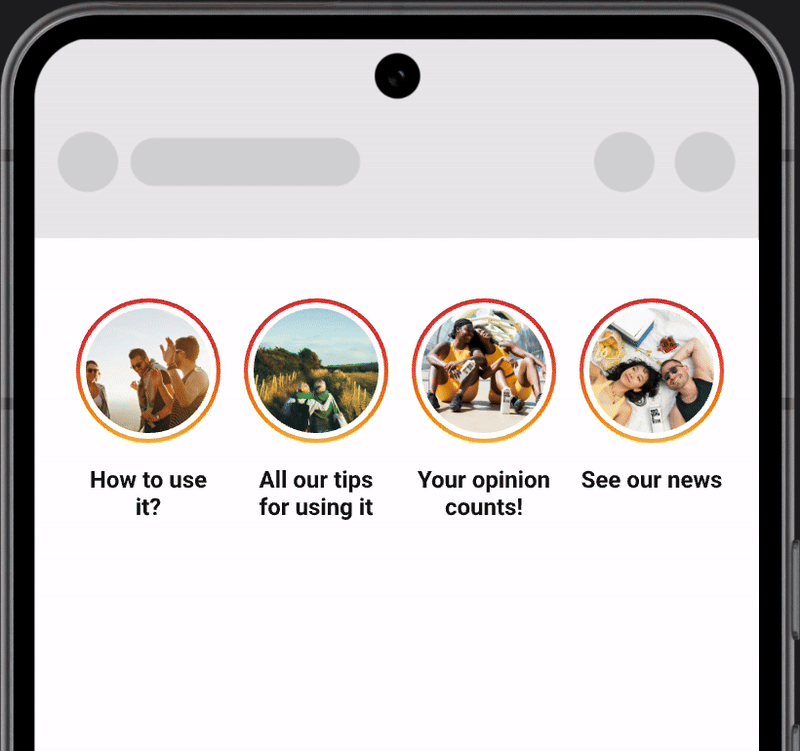
Player Standalone Mode
If you want to open the player to display stories following an action (event, button click, etc.), you can use the player in standalone mode. Simply call a method to open the player as follows :
JOINStories.startPlayer(context: Context, alias: "<your_story_alias>")
Additional Methods
Max stories
You can customise the number of stories you want to display in the trigger by using the maxStories
parameter at initialization (by default it will display all stories):
trigger.init = JOINStoriesInit(alias = "<your_story_alias>", maxStories = Int)
Dismiss Player Manually
If you want to dismiss the player manually, use can use the dismissPlayer
method :
trigger.dismissPlayer()
JOINStories.dismissPlayer()
Reorder stories already viewed
Stories can be reordered at the end of the widget if they have already been viewed in their entirety. To do this, simply set the reorderedReadStories
parameter to true and the change will take effect.
val config = BubbleConfiguration(reorderedReadStories = true)
...
val config = CardGridConfiguration(reorderedReadStories = true)
...
val config = CardListConfiguration(reorderedReadStories = true)
Refresh widget manually
You can manually fetch stories from the widget (as after a pull-to-refresh) using the refresh method in the widget classes.
val bubble: BubbleTriggerView = BubbleTriggerView(context)
bubble.refresh()
...
val card: CardTriggerView = CardTriggerView(context)
card.refresh()
Set Up Listener
The JOIN Stories SDK exposes a listener JOINStoriesListener
who notifies the application when an event occurs. You can register the listener using the following code example and then override its functions to learn about specific events, which will be explained in the next sections.
bubbleTriggerView.joinListener = object : JOINStoriesListener {
...
}
//OR
JOINStories.startPlayer(context, "<your_story_alias>", listener = object : JOINStoriesListener {
...
})
onTriggerFetchSuccess
This event tells you that the trigger has completed its network operations and that there are stories to display.
Returns the number of items displayed.
override fun onTriggerFetchSuccess(itemCount: Int) {}
onTriggerFetchEmpty
This event tells you that the trigger has completed its network operations and that there are no stories to display. This can happen if the add alias has no stories configured or if there are no stories already stored locally in offline mode.
override fun onTriggerFetchEmpty() {}
onTriggerFetchError
This event tells you that the trigger has completed its network operations and had a problem while fetching your stories.
override fun onTriggerFetchError(errorMessage: String) {}
onPlayerFetchSuccess
This event tells you that the player has completed its operations (network or local) and that there are stories to display.
override fun onPlayerFetchSuccess() {}
onPlayerLoaded
This event tells you that the player is loaded and playing the stories
override fun onPlayerLoaded() {}
onPlayerFetchError
This event tells you that the trigger has completed its operations (network or local) and had a problem while fetching your stories.
override fun onPlayerFetchError(errorMessage: String) {}
onPlayerDismissed
This event tells you that the player has disappeared and how. It can be automatic when all the stories have finished being viewed without any user interaction. It can be manual when it's the user who makes the player disappear or via an event implemented in the application.
override fun onPlayerDismissed(type: OnDismissState) {
when(type) {
OnDismissState.OnDismissAuto -> {}
OnDismissState.OnDismissManual -> {}
}
}
onContentLinkClick
This event tells you that a user has clicked on a CTA in the player. It indicates the CTA link in parameters and you can customize the behaviour based on this link (deeplink, path, https, etc...).
The method should return true if the app manages the link click behaviour, and false if the app does not want to catch the link provided. If a false is returned, the SDK will try to open the link in the default browser of the device. By default, the method return false.
fun onContentLinkClick(link: String): Boolean {
return false
}
Updated 14 days ago
Dont hesitate to continue