Initial SDK Setup
SDK Installation
Our JOIN Stories React Native SDK is available via npm. You can install package like this :
npm install @join-stories/react-native-widgets
yarn add @join-stories/react-native-widgets
pnpm add @join-stories/react-native-widgets
Android compatibility
JOIN Stories SDK targets Android API level 21 (Android 5.0, Lollipop) or higher.
iOS compatibility
JOIN Stories SDK targets iOS 12 or higher.
SDK Initialization
TeamId and widget alias
You will need your team id and the widgets' alias you want to integrate. You can find both of them in the Integration tab of your widget mobile on studio.
To create a widget, check the documentation. Make sure to choose Mobile app environment.
Before using any feature offered by the SDK, you have to initialize it. At the root of your App, you need to do this:
import { JoinStories } from "@join-stories/react-native-widgets";
export default function App() {
useEffect(() => {
JoinStories.init("<your_join_team_id>");
}, []);
return <MyApp />;
}
Init SDK with an api key
You can initialize the SDK with an api key supplied by the JOIN technical team. You can add it to the SDK using the initWithApiKey function :
import { JoinStories } from "@join-stories/react-native-widgets";
export default function App() {
useEffect(() => {
JoinStories.initWithApiKey("<your_join_team_id>", "<api-key>");
}, []);
return <MyApp />;
}
Add Component
Warning
When integrating, you need to be aware of the widget's lifecycle, as the widget may send request several times during use if it is destroyed and recreated, in the case of a dynamic list.
Destroying and recreating the view will generate a loaded widget, which will be counted towards your pricing
After SDK initialization, you can display your widget anywhere you like, for example on HomePage:
import { JoinStoriesView } from '@join-stories/react-native-widgets';
export default function Home() {
return (
<SafeAreaView>
<Text>Welcome !</Text>
<JoinStoriesView alias="<your_join_alias>" />
</SafeAreaView>)
}
Good job !
You have integrated your first component and are ready to see stories in your application.
Customization
JoinStoriesView is the default view (or trigger). It displays stories in a bubble format like Instagram. You can use other formats like Card. For more info, see UI Customizations.
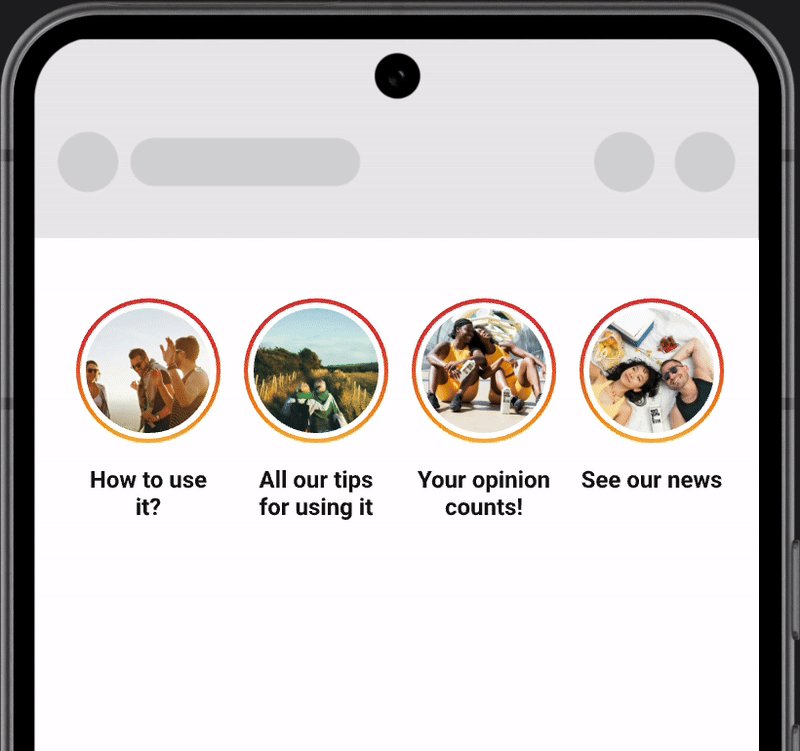
Player Standalone Mode
If you don't need to display bubbles (or cards) and just want to open the player following an action (event, button click, etc.), you can use the player in standalone mode. Simply call a method to open the player as follows :
import { JoinStories } from "@join-stories/react-native-widgets";
export default function Home() {
const openPlayer = () => {
JoinStories.startStandalonePlayer({ alias: '<your_join_alias>' });
}
return (
<SafeAreaView>
<Button title="Open player" onPress={openPlayer} />
</SafeAreaView>)
}
It will open on the first story of the given widget.
Dismiss Player Manually
If you want to dismiss the player manually, you can use the dismissPlayer
method :
import { JoinStories } from "@join-stories/react-native-widgets";
export default function Home() {
const dismissPlayer = () => {
JoinStories.dismissPlayer();
}
return (
<SafeAreaView>
<Button title="Dismiss player" onPress={dismissPlayer} />
</SafeAreaView>)
}
Info
This method can also be used to dismiss a player opened via a bubble or card component.
Refresh widget manually
You can manually fetch stories from the widget (as after a pull-to-refresh) using the refresh method in the widget classes.
import { Text, TouchableOpacity } from 'react-native';
import { JoinStoriesView, JoinStoriesViewRef} from '@join-stories/react-native-widgets';
import { useRef } from 'react';
export default function Page() {
const storiesViewRef = useRef<JoinStoriesViewRef>(null);
const handleRefresh = () => {
storiesViewRef.current?.refresh();
};
return (
<TouchableOpacity onPress={handleRefresh}>
<Text>Refresh Stories</Text>
</TouchableOpacity>
<JoinStoriesView
ref={storiesViewRef}
alias="alias"
/>
);
}
Set Up Player Listener
Add Listener
The JOIN Stories SDK exposes a listener who notifies the application when an event occurs on your widget and player. You can register the listener using the following code example.
import { JoinStoriesView, JoinStories } from '@join-stories/react-native-widgets';
export default function Home() {
useEffect(() => {
const playerListener = JoinStories.addPlayerListener((event) => {
console.log('event received', event);
});
return () => {
playerListener.remove();
};
}, [])
return (
<SafeAreaView>
<Text>Welcome !</Text>
<JoinStoriesView alias="<your_join_alias>" />
</SafeAreaView>)
}
Events
Here is a list of the events you can receive:
EventType | Payload | Description |
---|---|---|
TriggerFetchSuccess | itemCount (number): number of stories that were retrieved. | This event tells you that the trigger has completed its network operations and that there are stories to display. |
TriggerFetchEmpty | This event tells you that the trigger has completed its network operations and that there are no stories to display. This can happen if the add alias has no stories configured or if there are no stories already stored locally in offline mode. | |
TriggerFetchError | message (string): error message | This event tells you that the trigger has completed its network operations and had a problem while fetching your stories. |
PlayerFetchSuccess | This event tells you that the player has completed its operations (network or local) and that there are stories to display. | |
PlayerLoaded | This event tells you that the player is loaded and playing the stories | |
PlayerFetchError | message (string): error message | This event tells you that the trigger has completed its operations (network or local) and had a problem while fetching your stories. |
PlayerDismissed | state ('auto' | 'manual') | This event tells you that the player has disappeared and how. It can be automatic when all the stories have finished being viewed without any user interaction. It can be manual when it's the user who makes the player disappear or via an event implemented in the application. |
ContentLinkClick | link (string): CTA link | This event tells you that a user has clicked on a CTA in the player. By default, it will try to open a web browser. |
If you are using typescript, you can also import and use the PlayerEvent
type.
Prevent default behavior
Some events are followed by specific actions (i.e ContentLinkClick
tries to open the link in a browser). To prevent this happening, you can call event.preventDefault()
. See :
import { JoinStoriesView, JoinStories } from '@join-stories/react-native-widgets';
export default function Home() {
useEffect(() => {
const playerListener = JoinStories.addPlayerListener((event) => {
event.preventDefault()
// implement your own logic
});
return () => {
playerListener.remove();
};
}, [])
return (
<SafeAreaView>
<Text>Welcome !</Text>
<JoinStoriesView alias="<your_join_alias>" />
</SafeAreaView>)
}
Updated 21 days ago